GoLang Tutorial
GoLang is super fast server side language for web framework and Restful API. It's must faster than other traditional language and supports concurrency.
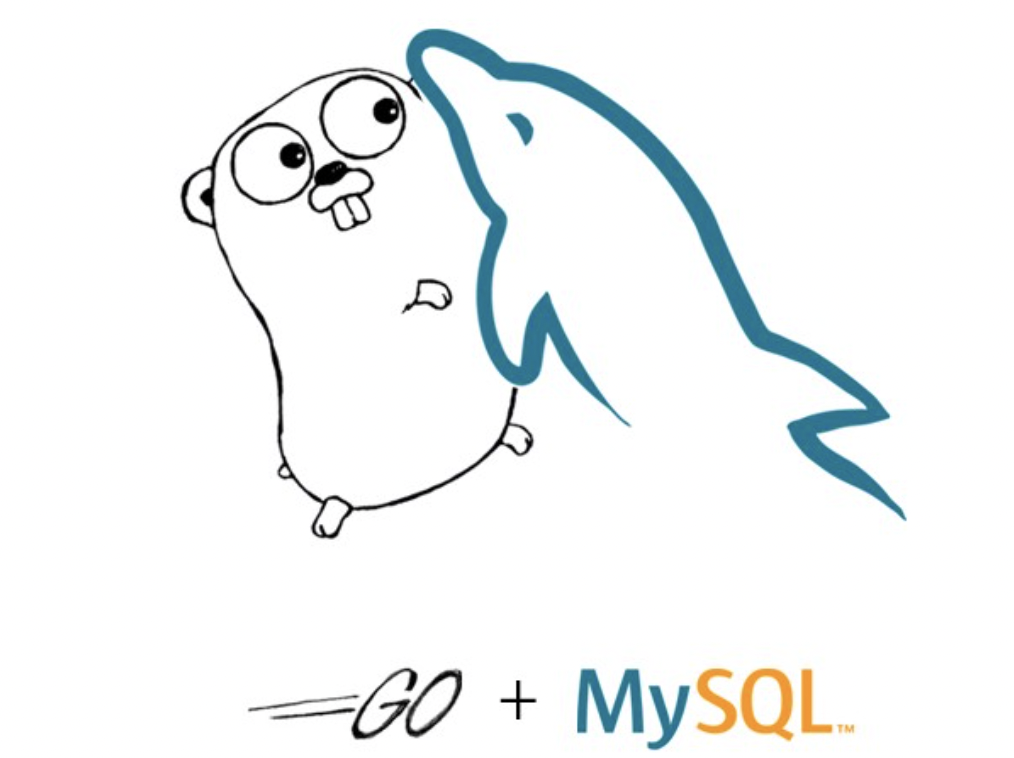
Here we will see we can connect mysql with GoLang.
To connect mysql with GoLang
First go to your root folder of your project and run the command
go get github.com/go-sql-driver/mysql
It should download the mysql package
2. After that import mysql like below
import ( "database/sql" _ "github.com/go-sql-driver/mysql" )
3. Then in your main function of Go program, you can initialize it like below
db, err := sql.Open("mysql", "root:123456@tcp(127.0.0.1:3306)/task_management") // if there is an error opening the connection, handle it if err != nil { log.Print(err.Error()) } defer db.Close() var tag Tasks // Execute the query err = db.QueryRow("SELECT id, task_name FROM tasks where id = ?", 2).Scan(&tag.ID, &tag.TaskName) if err != nil { panic(err.Error()) // proper error handling instead of panic in your app } log.Println(tag.ID) log.Println(tag.TaskName)
In the above code my password is 12345 and user name is root. And database name is task_managment. You need to change them as per your need..
In the db.QueryRow you need to change the the table name based on your needs.