GoLang Tutorial
GoLang is super fast server side language for web framework and Restful API. It's must faster than other traditional language and supports concurrency.
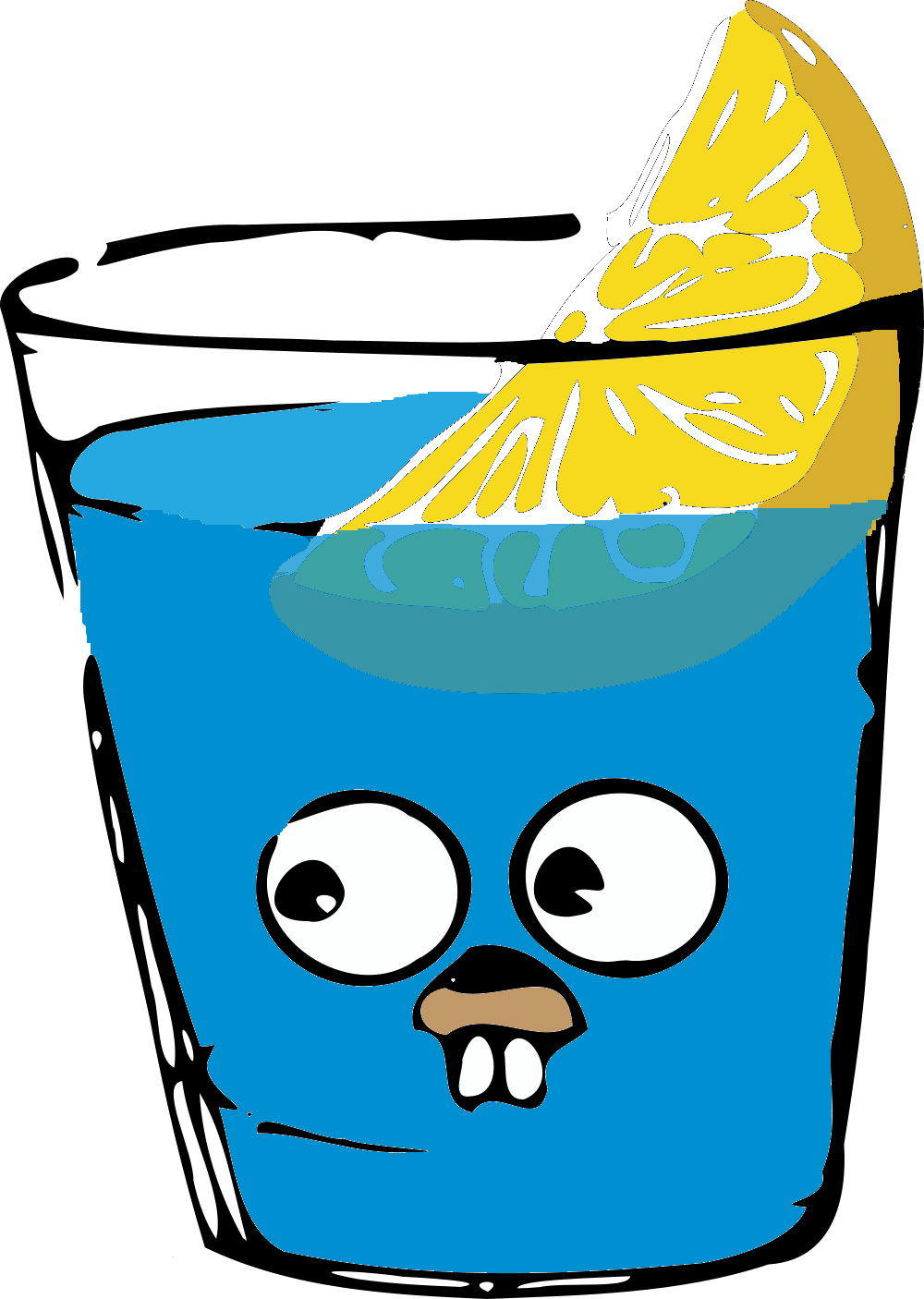
How to get the query params in Go Gin
To get the query params in GoLang Gin you have to use the below syntax inside your route callback function.
c.Request.Url.Query().Get("param")
The above statement will return you a string. Inside the param you need to mention your parameters you want to catch
The complete code is below
package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/query", func(c *gin.Context) { first := c.Request.URL.Query().Get("first") //<---- here! c.String(http.StatusOK, "First is "+first+"\n") second := c.Request.URL.Query().Get("second") c.String(http.StatusOK, "Second is "+second+"\n") }) r.Run(":8080") // listen and serve on 0.0.0.0:8080 }
So if your request is like
http://localhost:8080/query?first=123&second=abc
Then you will retreive 123 and abc